Verify SDK for Web
In this comprehensive guide, we'll navigate you through the essential steps to kickstart your journey with the Synaps Verify SDK on Web platform.
Add package
- npm
- yarn
npm install @synaps-io/verify-sdk
yarn add @synaps-io/verify-sdk
Properties
sessionId
string
Unique verification session identifier
mode
string
- modal - Opens a modal using
Synaps.show()
- embed - Load directly into your div
containerId
onFinish()
callback (optional)
Callback when the user finishes the verification process
onClose()
callback (optional)
Callback when the user closes the verification process
service
string (optional)
- individual for KYC (default)
- corporate for KYB
containerId
string (optional)
required when using embed
mode
withFinishButton
boolean (optional)
When using embed
mode, add a finish button on the offboarding screen
lang
string (optional)
User language preference
- en - English
- fr - French
- de - German
- es - Spanish
- it - Italian
- ja - Japanese
- ko - Korean
- pt - Portuguese
- ro - Romanian
- ru - Russian
- tr - Turkish
- vi - Vietnamese
- zh-CN - Simplified Chinese
- zh-TW - Traditional Chinese
You can filter steps by Level thanks to the level=$LEVEL_ID
query parameter.
Web librairies
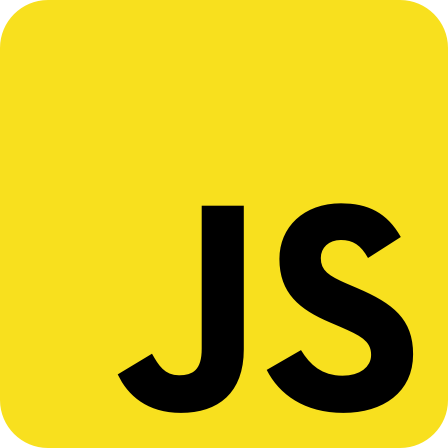
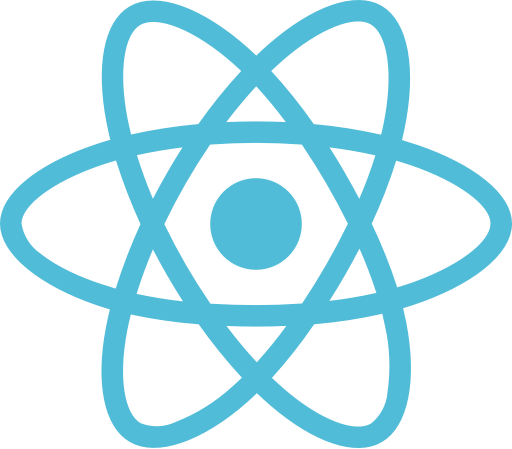
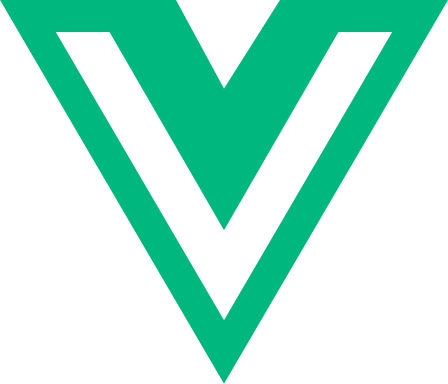
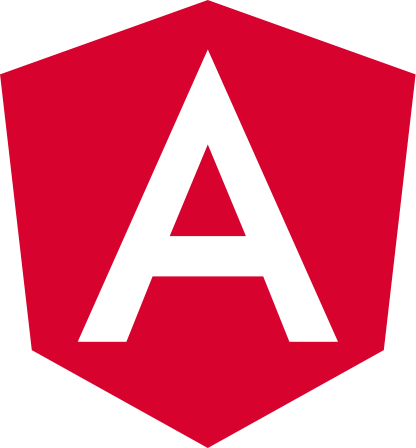
Javascript
1. Add Synaps element
There is two integration methods: modal or embed
modal: place a button and open the verification flow when user click on the button
embed: integrate directly the verification flow into your interface
2. Modal integration (verify with Synaps button)
Add the following element to your page to add the button.
- Modal HTML
- Modal javascript
- Modal Native HTML script
<button id="synaps-btn">Verify with Synaps</button>
import { Synaps } from "@synaps-io/verify-sdk";
document.querySelector("#synaps-btn").addEventListener("click", () => {
Synaps.show();
});
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
onFinish: () => {
alert("Verification finished");
},
mode: "modal",
});
<body>
<div>Hello World</div>
<button id="synaps-btn">Verify with Synaps</button>
<script type="module">
import { Synaps } from "https://cdn.synaps.io/sdk/verify.js/$VERSION";
document.querySelector("#synaps-btn").addEventListener("click", () => {
Synaps.show();
});
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
onFinish: () => {
alert("Verification finished");
},
mode: "modal",
});
</script>
</body>
Don't forget to replace $YOUR_SESSION_ID
with your own session id.
3. Embed integration
Add the following element to add the verification flow to your page.
- Embed HTML
- Embed javascript
- Embed Native HTML script
<div class="App">
<div style="width: 300px; height: 600px;" id="synaps-wrapper"></div>
</div>
import { Synaps } from "@synaps-io/verify-sdk";
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
onFinish: () => {
alert("Verification finished");
},
containerId: "synaps-wrapper",
mode: "embed",
});
<body>
<div>Hello World</div>
<div class="App">
<div style="width: 300px; height: 600px" id="synaps-wrapper"></div>
</div>
<script type="module">
import { Synaps } from "https://cdn.synaps.io/sdk/verify.js/$VERSION";
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
onFinish: () => {
alert("Verification finished");
},
containerId: "synaps-wrapper",
mode: "embed",
});
</script>
</body>
Don't forget to replace $YOUR_SESSION_ID
with your own session id.
React
- Add package
- Read properties
- Test examples
Verify example with Modal mode
import { Synaps } from "@synaps-io/verify-sdk";
import { useEffect } from "react";
const Modal = () => {
useEffect(() => {
// Prevent multiple initializations with react strict mode
// https://react.dev/learn/synchronizing-with-effects#fetching-data
let init = true;
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
onFinish: () => {
alert("Verification finished");
},
mode: "modal",
});
return () => {
init = false;
};
}, []);
const handleOpen = () => {
Synaps.show();
};
return (
<div className="App">
<button onClick={handleOpen}>Start verification</button>
</div>
);
};
export default Modal;
Verify example with Embed mode
import { Synaps } from "@synaps-io/verify-sdk";
import { useEffect } from "react";
const Embed = () => {
useEffect(() => {
// Prevent multiple initializations with react strict mode
// https://react.dev/learn/synchronizing-with-effects#fetching-data
let init = true;
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
onFinish: () => {
alert("Verification finished");
},
mode: "embed",
});
return () => {
init = false;
};
}, []);
return (
<div className="App">
<div style={{ width: 300, height: 600 }} id={"synaps-wrapper"} />
</div>
);
};
export default Embed;
Vue
- Add package
- Read properties
- Test examples
Verify example with Modal mode
<script>
import {Synaps} from "@synaps-io/verify-sdk";
import {defineComponent} from "vue";
export default defineComponent({
mounted() {
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
mode: "modal",
onFinish: () => {
alert("Verification finished")
}
})
},
methods: {
handleOpen() {
Synaps.show();
}
}
})
</script>
<template>
<div>
<button v-on:click="this.handleOpen()">
Start verification
</button>
</div>
</template>
Verify example with Embed mode
<script>
import { Synaps } from "@synaps-io/verify-sdk";
import { defineComponent } from "vue";
export default defineComponent({
mounted() {
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
mode: "embed",
onFinish: () => {
alert("Verification finished")
}
})
},
})
</script>
<template>
<div>
<div style="width: 300px; height: 600px" id="synaps-wrapper"></div>
</div>
</template>
Svelte
- Add package
- Read properties
- Test examples
Verify example with Modal mode
<script lang="ts">
import {Synaps} from "@synaps-io/web-sdk";
import {onMount} from "svelte";
onMount(() => {
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
mode: "embed",
onFinish: () => {
alert("Verification finished")
}
})
})
</script>
<main>
<div style="width: 300px; height: 600px" id="synaps-wrapper"/>
</main>
Verify example with Embed mode
<script lang="ts">
import {Synaps} from "@synaps-io/web-sdk";
import {onMount} from "svelte";
onMount(() => {
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
mode: "modal",
onFinish: () => {
alert("Verification finished")
}
})
})
function handleOpen() {
Synaps.show()
}
</script>
<main>
<button on:click={handleOpen}>
Start verification
</button>
</main>
Angular
- Add package
- Read properties
- Test examples
Verify example with Modal mode
- app.component.html
- app.component.ts
<div>
<button (click)="handleOpen()">Start verification</button>
<div id="synaps-wrapper"></div>
</div>
import { Component } from "@angular/core";
import { Synaps } from "@synaps-io/verify-sdk";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
handleOpen = () => {
Synaps.show();
};
ngOnInit() {
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
onFinish: () => {
alert("finish");
},
});
}
}
Verify example with Embed mode
- app.component.html
- app.component.ts
<div>
<button (click)="handleOpen()">Start verification</button>
<div id="synaps-wrapper"></div>
</div>
import { Component } from "@angular/core";
import { Synaps } from "@synaps-io/verify-sdk";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
ngOnInit() {
Synaps.init({
sessionId: "$YOUR_SESSION_ID",
onFinish: () => {
alert("finish");
},
});
}
}